In this part of Scala blog series we’ll look at Traits, which is one of the great feature in Scala.
The main intention to use Traits is to implement reuse code segments in Scala. It can be thought of as interface that encapsulates method and field definitions. But it’s not necessary to provide implementation for all/some methods that the trait defines. Therefore, the implementation can be reuse to expand the class functionality by mixing them. Unlike class inheritance, in which one class must inherit from just one superclass, a class can extends and mix with any number of traits. Hence, we can also think the concept as multiple inheritance like in C# language.
If the methods don’t need arguments we can just declare the declaration name for the traits.
trait Fish {
def eat
def swim
}
If method requires parameter, So the implementation goes as usual.
trait Animal {
def eat(whatEats: String)
}
When extending one trait, use extends.
class Dolphen extends Fish{ ... }
More than one traits, use extends for the class/first trait and with for subsequent traits.
class Dolphen extends Fish with Trait1 with Trait2 { ... }
If the methods are not implemented in the traits, it’s a must that we should implement the abstract traits methods.
class Dolphen extends Fish{
def eat { //implementation }
def swim { //implementation }
}
Lets take a general example and try to understand much more clearly. See the following class diagram (Figure 1) that shows hierarchy of Animals.
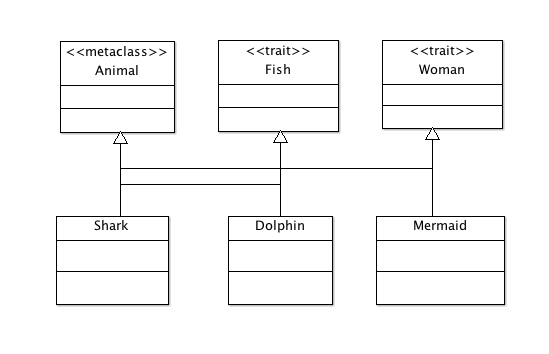
The class hierarchy diagram clearly shows that we are maintaining our code segments separately and it allows scaling up/down since everything is independent.
Look at our Shark, Dolphin and Mermaid with a comprehensive analysis mind. I’m sure you will be able find out similar things in between each animal and the very unique behaviour/attribute of each animal. So to build up a animal we can use the traits to do the mixing to get the multiple behaviours/attributes. The following below code snippet disclose the features of traits.
abstract class Animal {
def speak
}
trait Fish {
def eat
def swim
def speak
}
trait Woman {
def longHair { println("I have long hair") }
}
class Dolphin extends Fish {
override def eat: Unit = { println("I eat herring..") }
override def swim: Unit = { println("I can swim in shallow water..")}
override def speak: Unit = { println("I can whistle..")}
def dance { println("I can dance..") }
}
class Shark extends Fish {
override def eat: Unit = ???
override def swim: Unit = ???
override def speak: Unit = ???
def kill { println("I can kill others..") }
}
class Mermaid extends Fish with Woman{
override def eat: Unit = ???
override def swim: Unit = ???
override def speak: Unit = ???
def sing { println("I can Sing..") }
}