Like in java and .net a class is a blue print for objects. Once we define class, we can create multiple objects from the class with the keyword new.
class Product {
// class definition goes here
}
//creating an object
new Product
Fields
class Product {
var price = 0 //Field
}
val soap = new Product
val chair = new Product
since field is var type after creating the object we can reassign with a different value to the field.
soap.price = 30
The image of the objects in memory might look like:Figure 1
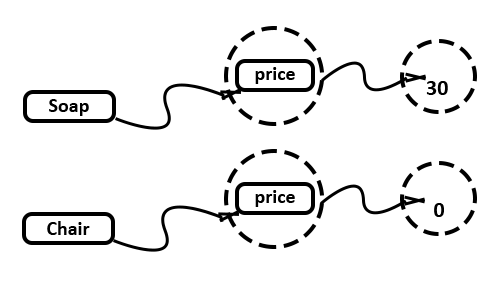
It’s obvious; soap object cannot be reinitialized because the objects given that they are vals, not vars.
// Won’t compile, because soap is a val
soap = new Product
“public” in Java, you simply say nothing in Scala
class Product {
private var price = 0
def add(b: Int): Unit = {
price += b
}
}
”semicolon” at the end of a statement is usually optional
var hello = “hello scala without semicolon”
But, a semicolon is required if you write multiple statements on a single line:
var hello = “hello scala with semicolon”; println(hello)
Singleton Object
Scala is more unique and easy to use language than java.
Scala cannot have static members
Scala has an easy way to define singleton object. Like we defining a class in scala we can easily define an object.
Instead of the keyword “class” we use the keyword “object”
object Product {
private var price = 0
def add(b: Int): Unit = {
price += b
}
}
Hope you understood about classes and objects in scala. Next we will look into functional objects and methods.