Defining variables
Scala has two kinds of variables, vals and vars. A val is similar to a final variable in Java. Once initialized, a val can never be reassigned. A var, its more likely var variables in C#. A var can be reassigned throughout its lifetime. Here’s a val definition: Figure 1
val msg = "Hello Scala World!"
/*
Compilation error
Can't assign value to "val"
* */
msg = "Scala Confusing"
var msg = "Hello Scala World!"
/*
Can assign value to "var"
* */
msg = "Scala <3"
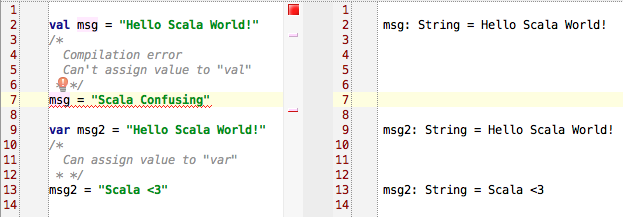
Scala Functions
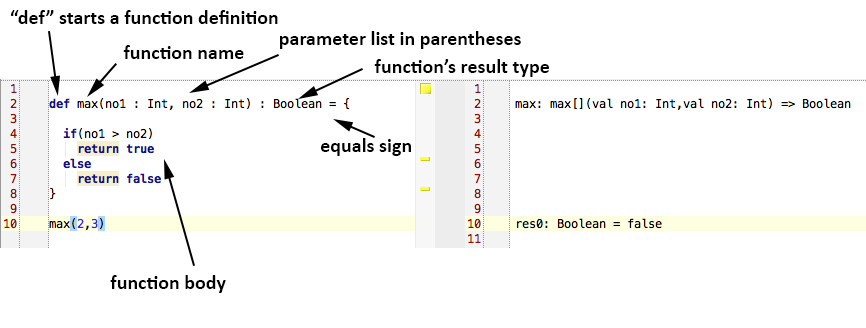
Loops with while and for decision if
var args = Array(-1,3,4,-5) //Array
var i = 0
while (i < args.length) { //While loop
if (i != 0) //If condition
print(" ")
print(args(i))
i += 1
}
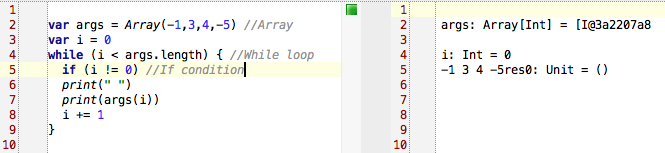
Iterate with foreach and for
var args = Array(-1,3,4,-5) //Array
args.foreach(arg => println(arg))
for (arg <- args)
println(arg)

Array
val greetStrings = new Array[String](3)
greetStrings(0) = "Hello"
greetStrings(1) = ", "
greetStrings(2) = "world!\n"
for (i <- 0 to 2)
print(greetStrings(i))

Operations
Basically all operations are methods call in scala
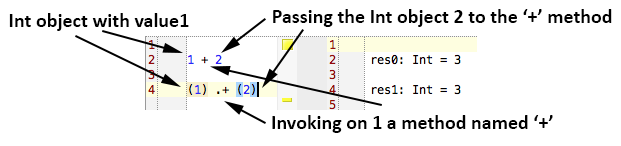
List
- Mutated (:::) Basically it’s like ‘Python Extends’. It will include two list and will return with a new list.
val l1 = List(1, 2, 3)
val l2 = List(1, 2)
val l3 = List(3, 4)
/* Mutated and created and
* Created a new list */
val l4 = l2 ::: l3
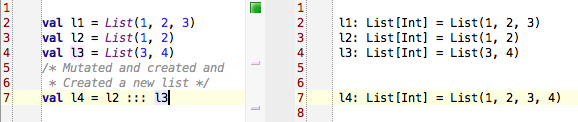
- Cons (::) Cons prepend a new element to the beginning of an existing list, and return the resulting list.
val l1 = List(1, 2, 3)
//Cons
val l2 = 0 :: l1

- Nil
val ls1 = List(1,2,3)
val ls2 = 1::2::3::Nil
println(s"New list with 'List', $ls1")
println(s"New list with 'Nil', $ls2")

To get more details on list check the scala api doc
Tuples
If you are a Python programmer you would have probably know about this container. It’s a very useful container object. Like lists, tuples are immutable, but unlike lists, tuples can contain different types of elements.
//Tuples
val pair = (99, "Scala <3")
println(pair)
println(pair._1)
println(pair._2)

Mutable and Immutable
Mutable and immutable are English words meaning “can change” and “cannot change” respectively. The meaning of the words is the same in the IT context; i.e.
- a mutable string can be changed, and
- an immutable string cannot be changed.
- For example, arrays are always mutable; lists are always immutable
Sets and Maps
//Set
var fishSet = Set("Gold Fish", "Angel Fish")
fishSet += "Piranha"
println(fishSet.contains("Cichlid"))
//Map
val romanNumbers = Map(
1 -> "I", 2 -> "II", 3 -> "III", 4 -> "IV", 5 -> "V"
)
println(romanNumbers(4))

Now that we have seen some scala code, you can try and have fun with scala ☺. In my next blog lets dive into more details on class and objects.